Nested if and Multi-Way if-else Statements
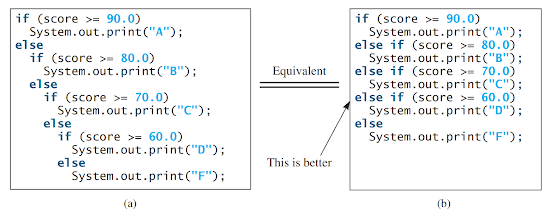
In Java, the standard output device is referred to as System.out, while the standard input device is referred to as System.in. By default, the output device is the display monitor, and the input device is the keyboard. To display information on the console, you can use the println method to output primitive values or strings.
Console input is not directly supported in Java, but you can utilize the Scanner class to create an object that can read input from System.in. The following line of code demonstrates how to create a Scanner object for this purpose:
Scanner input = new Scanner(System.in);
By using the syntax "new Scanner(System.in)", you instantiate a Scanner object. The line "Scanner input" declares a variable named "input" of type Scanner. The complete line "Scanner input = new Scanner(System.in)" creates a Scanner object and assigns its reference to the "input" variable.
Once you have the Scanner object, you can invoke its methods. Invoking a method on an object means asking the object to perform a specific task. To read a double value from the console, you can use the nextDouble() method as shown:
double radius = input.nextDouble();
This statement reads a number from the keyboard and assigns it to the variable "radius".
Let's go through the code step by step:
1. Importing the Scanner class:
The line `import java.util.Scanner;` imports the Scanner class from the `java.util` package. This allows us to use the Scanner class to read input from the console.
2. Defining the class:
The line `public class ComputeAreaWithConsoleInput` defines a public class named `ComputeAreaWithConsoleInput`.
3. Defining the main method:
The line `public static void main(String[] args)` defines the main method, which serves as the entry point for the program.
4. Creating a Scanner object:
The line `Scanner input = new Scanner(System.in);` creates a new Scanner object named `input`. This object is used to read input from the console.
5. Prompting the user for input:
The line `System.out.print("Enter a number for radius: ");` displays a prompt to the user, asking them to enter a number for the radius.
6. Reading user input:
The line `double radius = input.nextDouble();` reads a double value from the user using the Scanner object and assigns it to the variable `radius`.
7. Computing the area:
The line `double area = radius * radius * 3.14159;` calculates the area of the circle using the formula `radius * radius * 3.14159`.
8. Displaying the result:
The line `System.out.println("The area for the circle of radius " + radius + " is " + area);` prints the calculated area along with the provided radius to the console.
9. Closing the Scanner object:
The line `input.close();` closes the Scanner object to release system resources.
When you run this program, it prompts the user to enter a number for the radius. After the user provides the input, it calculates the area of the circle using the formula `radius * radius * 3.14159` and displays the result on the console. Finally, it closes the Scanner object to free up resources.
Note: The value of `3.14159` is an approximation of the mathematical constant π (pi) commonly used in geometry to calculate the area of a circle.
In programming, identifiers and variables play a crucial role in defining and manipulating data. They are fundamental concepts that allow programmers to create meaningful and reusable code. This topic will delve into the concepts of identifiers and variables, their characteristics, rules for naming them, and their usage in various programming languages.
1. Identifiers:
Identifiers are names given to entities in a program, such as variables, functions, classes, and methods. They act as unique identifiers or labels that distinguish one entity from another. Identifiers are used to refer to these entities and are essential for understanding and organizing code.
2. Naming Rules for Identifiers:
- Identifiers can consist of letters (uppercase or lowercase), digits, and underscores.
- They must start with a letter or an underscore. Digits cannot be the first character.
- Identifiers are case-sensitive, meaning "myVariable" and "myvariable" are considered different.
- They should be descriptive and meaningful, reflecting the purpose or nature of the entity they represent.
- It is advisable to follow naming conventions and style guidelines specific to the programming language or community.
3. Variables:
Variables are storage locations used to hold data during program execution. They provide a way to store and manipulate values that can change over time. Variables have a name (identifier) and a data type that determines the kind of values they can hold, such as numbers, strings, or objects.
4. Variable Declaration and Assignment:
Variables need to be declared before they can be used. The declaration specifies the identifier (variable name) and the data type it will hold. For example, in Java, you can declare an integer variable as follows: `int myVariable;`. The variable can then be assigned a value using the assignment operator (`=`), such as `myVariable = 10;`, or it can be declared and assigned in a single line: `int myVariable = 10;`.
5. Variable Scope:
Variables have a scope, which defines their visibility and accessibility within a program. The scope determines where a variable can be accessed and used. Variables can have local scope (limited to a specific block of code or function) or global scope (accessible throughout the entire program).
6. Variable Lifetime:
Variables also have a lifetime, which refers to the duration for which they exist in memory. Local variables are created when a block of code is entered and destroyed when the block is exited. Global variables, on the other hand, exist throughout the entire program's execution.
7. Variable Usage:
Variables are used for various purposes, such as storing input values, performing calculations, holding intermediate results, and storing output values. They provide a means to manipulate data, control program flow, and facilitate dynamic behavior in programs.
Understanding the concepts of identifiers and variables is essential for writing effective and readable code. By following proper naming conventions, choosing meaningful identifiers, and correctly using variables, programmers can create code that is easier to understand, maintain, and debug.
Comments
Post a Comment