Nested if and Multi-Way if-else Statements
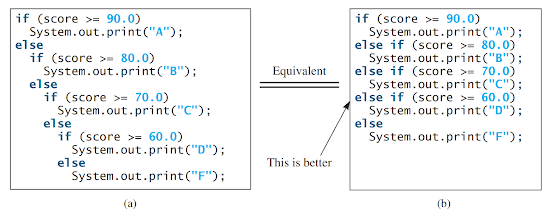
Each data type in Java has a specific range of values associated with it. When you declare a variable or constant, the compiler sets aside memory space based on its data type. Java offers eight primitive data types that cater to numeric values, characters, and Boolean values. In this section, we will focus on introducing numeric data types and the operators used with them.
Table-1 lists the six numeric data types, their ranges, and their storage sizes.
When it comes to floating-point numbers, Java provides two types: float and double. The double type is twice as large as the float type, and it is referred to as "double precision," while float is known as "single precision." Generally, it is advisable to use the double type because it offers higher precision and accuracy compared to the float type.
By now, you are familiar with utilizing the `nextDouble()` method from the `Scanner` class to read a double value from the keyboard. Additionally, you can employ the methods mentioned in Table -2 to read numeric values of different types, such as byte, short, int, long, and float. These methods enable you to retrieve input from the user in a specific numeric format according to your needs.
Here are examples for reading values of various types from the keyboard:
If you enter a value with an incorrect range or format, a runtime error would occur. For example, you enter a value 128 for line 3, an error would occur because 128 is out of range for a byte type integer.
The operators used with numeric data types encompass the basic arithmetic operations, which consist of addition (+), subtraction (-), multiplication (*), division (/), and remainder (%),as shoen in Table-3. These operators are employed to perform mathematical calculations on numeric values. The operands refer to the values that are manipulated or operated on by these operators.
The "%" operator, known as the remainder or modulo operator, returns the remainder resulting from division. The left operand represents the dividend, while the right operand represents the divisor. Thus, in expressions like 7 % 3, the result is 1; in 3 % 7, the result is 3; in 12 % 4, the result is 0; in 26 % 8, the result is 2; and in 20 % 13, the result is 7.
The modulo operator (%), although commonly used with positive integers, can also be applied to negative integers and floating-point values. The sign of the remainder depends on the sign of the dividend. For instance, in expressions like -7 % 3, the result is -1; in -12 % 4, the result is 0; in -26 % -8, the result is -2; and in 20 % -13, the result is 7.
The remainder operator is highly valuable in programming. It can be used to determine whether a number is even or odd by checking if the number % 2 equals 0 for even numbers and 1 for odd numbers. Additionally, it can be used to calculate future dates or determine specific days of the week. For example, if today is Saturday, adding 7 days will result in another Saturday. Similarly, if you and your friends plan to meet in 10 days, you can find out the day by evaluating an expression such as:
This will help you determine the day of the week in 10 days, considering the cyclical nature of the seven days in a week.
A literal refers to a constant value that is directly specified within a program's code. For instance, in the following statements:
int numberOfYears = 34;
double weight = 0.305;
The values 34 and 0.305 are examples of literals. An integer literal can be assigned to an integer variable as long as it can fit within the variable's range. If a literal is too large for the variable to hold, a compilation error occurs. For instance, the statement `byte b = 128` would cause a compilation error because 128 exceeds the range of values that can be stored in a variable of the byte type (which ranges from -128 to 127).
By default, an integer literal is assumed to be of the int type, which has a range between -2,147,483,648 and 2,147,483,647. To specify an integer literal as the long type, which has a larger range, you can append the letter L or l to the literal. For example, to represent the integer 2,147,483,648 in a Java program, you would write it as 2,147,483,648L or 2,147,483,648l. This is necessary because the value exceeds the range of the int type. The letter L is preferred to use, as the lowercase letter l can easily be mistaken for the digit 1.
Floating-point literals in Java are represented by numbers with a decimal point. By default, a floating-point literal is considered to be of the double type. For example, the value 5.0 is interpreted as a double value, not a float value. To explicitly specify a number as a float, you can append the letter f or F to the literal. Similarly, to specify a number as a double, you can append the letter d or D. For instance, you can use 100.2f or 100.2F to denote a float number, and 100.2d or 100.2D to denote a double number.
It is important to note that a float value typically has 7 to 8 significant digits, meaning the number of digits that contribute to its precision, while a double value has 15 to 17 significant digits. This difference in precision allows double values to represent more precise decimal numbers compared to float values.
Floating-point literals in scientific notation represent numbers in the form of a * 10^b, where 'a' is the significand and 'b' is the exponent. For instance, the scientific notation for 123.456 is 1.23456 * 10^2, and the scientific notation for 0.0123456 is 1.23456 * 10^-2.
In Java, a special syntax is used to represent numbers in scientific notation. For example, the number 1.23456 * 10^2 can be written as 1.23456E2 or 1.23456E+2, where 'E' (or 'e') represents the exponent. Similarly, the number 1.23456 * 10^-2 can be written as 1.23456E-2.
The letter 'E' (or 'e') can be in either lowercase or uppercase and serves as a shorthand notation to represent the exponent part of the scientific notation.
Comments
Post a Comment