Nested if and Multi-Way if-else Statements
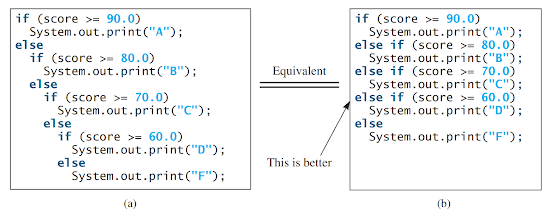
Operator precedence and associativity in Java determine the order in which operators are evaluated in an expression. Operator precedence defines which operators have higher priority over others, while associativity determines the order in which operators of the same precedence are evaluated.
Java follows a set of rules for operator precedence and associativity, which are as follows:
1. Parentheses: Operators enclosed in parentheses `()` have the highest precedence. Expressions inside parentheses are evaluated first.
2. Postfix operators: Postfix operators like `++` and `--` have the highest precedence after parentheses. They are evaluated from left to right.
3. Unary operators: Unary operators such as `++`, `--`, `+`, `-`, `!`, and `~` are evaluated next. They have a right-to-left associativity.
4. Multiplicative operators: Multiplication `*`, division `/`, and remainder `%` operators have higher precedence than additive operators. They are evaluated from left to right
5. Additive operators: Addition `+` and subtraction `-` operators are evaluated after multiplicative operators. They are evaluated from left to right.
6. Shift operators: Shift operators such as `<<`, `>>`, and `>>>` are evaluated next. They have a left-to-right associativity.
7. Relational operators: Relational operators like `<`, `>`, `<=`, and `>=` are evaluated after shift operators. They are evaluated from left to right.
8. Equality operators: Equality operators `==` and `!=` are evaluated after relational operators. They are evaluated from left to right.
10. Bitwise exclusive OR (XOR) operator: The bitwise XOR `^` operator is evaluated after the bitwise AND operator. It is evaluated from left to right.
11. Bitwise OR operator: The bitwise OR `|` operator is evaluated after the bitwise XOR operator. It is evaluated from left to right.
12. Logical AND operator: The logical AND `&&` operator is evaluated after the bitwise OR operator. It is evaluated from left to right.
13. Logical OR operator: The logical OR `||` operator has the lowest precedence among the binary operators. It is evaluated from left to right.
14. Conditional operator: The conditional (ternary) operator `? :` has the lowest precedence overall. It is evaluated from left to right.
When multiple operators of the same precedence are present in an expression, the associativity determines the order of evaluation. Left-associativity means the operators are evaluated from left to right, while right-associativity means they are evaluated from right to left.
Understanding operator precedence and associativity is crucial for writing correct and predictable expressions in Java. It helps ensure that the operations are performed in the intended order.
Comments
Post a Comment