Nested if and Multi-Way if-else Statements
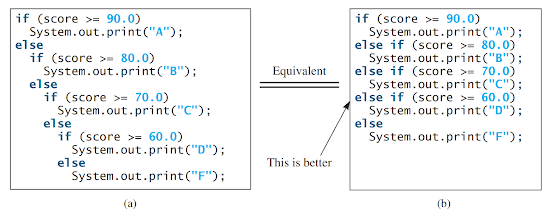
Floating-point numbers can be converted into integers using explicit casting. When performing binary operations with operands of different types, Java automatically converts the operand with the smaller range to the type with the larger range. For example, if you multiply an integer (3) with a floating-point number (4.5), Java will convert the integer to a floating-point value (3.0) before performing the multiplication. So, 3 * 4.5 is equivalent to 3.0 * 4.5.
n Java, you can always assign a value to a numeric variable that supports a larger range of values. For example, you can assign a long value to a float variable. However, assigning a value to a variable with a smaller range requires explicit type casting. Type casting is an operation that converts a value from one data type to another. Widening a type refers to casting a type with a smaller range to a type with a larger range, while narrowing a type refers to casting a type with a larger range to a type with a smaller range. Java automatically widens a type, but you must explicitly narrow a type.
To cast a type, you use the syntax of specifying the target type in parentheses, followed by the variable's name or the value to be cast.
For example, the statement
`System.out.println((int) 1.7);`
will display 1. When a double value is cast into an int value, the fractional part is truncated.
In the statement
`System.out.println((double) 1 / 2);`
the result will be 0.5 because 1 is cast to 1.0 first, and then 1.0 is divided by 2.
However, in the statement
`System.out.println(1 / 2);`
the result will be 0 because both 1 and 2 are integers, and the resulting value of the division should also be an integr.
Caution:
Casting is necessary when assigning a value to a variable of a smaller type range, such as assigning a double value to an int variable. If casting is not used in such situations, a compile error will occur. However, it is important to exercise caution when using casting, as it may result in loss of information and potentially lead to inaccurate results.
Note:
Casting does not change the original variable. For example, in the following code:
- double d = 4.5;
- int i = (int) d; // i becomes 4, but d remains 4.5
In Java, an augmented expression of the form `x1 op= x2` is implemented as `x1 = (T) (x1 op x2)`, where `T` is the type of `x1`. Therefore, the following code is correct:
The statement `sum += 4.5` is equivalent to `sum = (int) (sum + 4.5)`.
Explicit casting must be used when assigning a variable of type `int` to a variable of type `short` or `byte`. For example, the following statements will result in a compile error:
However, if the integer literal falls within the permissible range of the target variable, explicit casting is not needed when assigning an integer literal to a variable of type `short` or `byte`.
Comments
Post a Comment