Nested if and Multi-Way if-else Statements
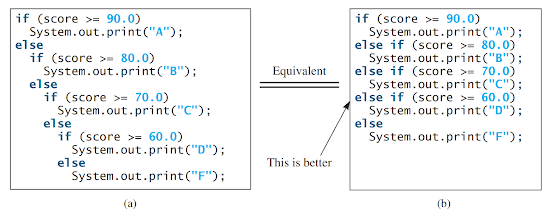
An assignment statement designates a value for a variable. An assignment statement can be used as an expression in Java.
Once you declare a variable, you have the ability to give it a value by utilizing an assignment statement. In Java, the assignment operator is represented by the equal sign (=). The structure for assignment statements follows this syntax:
variable = expression;
An expression denotes a computation that involves values, variables, and operators, which, when combined, yield a result. Let's examine the subsequent code as an illustration:
int y = 1; // Assign the value 1 to the variable y
double radius = 1.0; // Assign the value 1.0 to the variable radius
int x = 5 * (3 / 2); // Assign the value of the expression to x
x = y + 1; // Assign the sum of y and 1 to x
double area = radius * radius * 3.14159; // Compute the area
You can include a variable within an expression, and the variable can be used on both sides of the assignment operator (=). For instance,
x = x + 1;
In this assignment statement, the outcome of x + 1 is assigned to x. If x holds the value 1 before executing this statement, it will become 2 after the statement is executed.
To assign a value to a variable, you must place the variable name to the left of the assignment operator. Consequently, the following statement is incorrect:
1 = x; // Incorrect
In the Java programming language, an assignment statement can be seen as an expression that produces a value to be assigned to the variable located on the left side of the assignment operator. Due to this characteristic, an assignment statement is often referred to as an assignment expression. Let's illustrate this with an example:
System.out.println(x = 1);
This line of code is functionally equivalent to:
x = 1;
System.out.println(x);
When a value needs to be assigned to multiple variables simultaneously, you can employ the following syntax:
i = j = k = 1;
This statement can be interpreted as:
k = 1;
j = k;
i = j;
Thus, the value 1 is assigned to variable k, then the value of k is assigned to variable j, and finally, the value of j is assigned to variable i.
Comments
Post a Comment