Nested if and Multi-Way if-else Statements
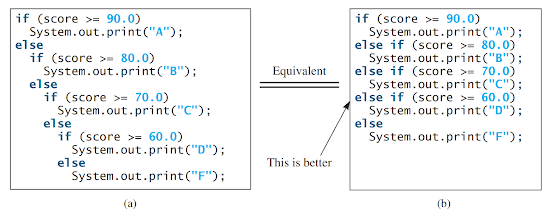
Programming style refers to the conventions and guidelines followed while writing code. A consistent and readable programming style not only improves code maintainability but also enhances collaboration among developers. Here are some key aspects of programming style:
1. Indentation and Formatting: Use consistent indentation (e.g., four spaces) to improve code readability. Properly format code by following conventions for line breaks, spacing, and alignment.
2. Naming Conventions: Choose meaningful and descriptive names for variables, functions, and classes. Use camelCase or snake_case for variable and function names, and capitalize class names (e.g., `myVariable`, `calculate_area()`).
3. Comments: Include comments to explain the code's logic, clarify complex algorithms, and provide context. Comments should be concise, relevant, and kept up to date.
4. Modularity and Readability: Break down complex tasks into smaller functions or methods. This promotes code reusability, readability, and easier debugging.
5. Consistency: Follow consistent patterns and conventions throughout the codebase. Use the same naming conventions, formatting, and style across all files and modules.
Documentation is an essential aspect of software development. It helps developers, maintainers, and users understand the codebase and its functionalities. Here are some types of documentation commonly used in programming:
1. Code Comments: Include comments within the code to explain specific sections, algorithms, or complex logic. Comments should be concise, informative, and placed strategically to enhance understanding.
2. Function and Method Documentation: Document functions and methods using docstrings, which provide information about their purpose, parameters, return values, and any exceptions they may raise. Tools like Sphinx can generate API documentation from docstrings.
3. Readme Files: Write a Readme file that provides an overview of the project, installation instructions, usage examples, and any additional information for developers or users.
4. Tutorials and Guides: Create tutorials or guides that explain how to use the software, provide examples, and demonstrate best practices.
5. API Documentation: For libraries or frameworks, generate API documentation that describes available classes, methods, parameters, and return values. Tools like Javadoc, Doxygen, or Sphinx can assist in generating comprehensive API documentation.
Errors are an inevitable part of software development. Understanding and effectively handling errors is crucial to creating robust and reliable applications. Here are some key points related to errors:
2. Exception Handling: Use exception handling mechanisms to catch and handle errors gracefully. This helps prevent program crashes and allows for error recovery or alternative actions.
3. Logging: Implement logging to record error messages, warnings, and other relevant information during program execution. Logging aids in troubleshooting and identifying issues in production environments.
4. Testing and Debugging: Thoroughly test the code and utilize debugging tools to identify and resolve errors. Automated testing frameworks, unit tests, and integration tests help detect errors early in the development process.
5. Error Messages: Provide clear and informative error messages that help users understand what went wrong and how to resolve the issue. Avoid cryptic error messages that make troubleshooting difficult.
By following good programming style, writing comprehensive documentation, and effectively handling errors, developers can create high-quality software that is maintainable, understandable, and robust. These practices contribute to the overall success and longevity of software projects.
Comments
Post a Comment